Notes from the Dawn of Time #8:
Inheritance Coda
by Richard Bartle
December 12, 2001
There are a few things about inheritance that I really ought to explain for the benefit of people planning on looking at my suggestions in detail. I wont go into the theory behind these this time or it would take another 3 articles. Instead, Ill just give you the solutions I use.
Pattern-Matching Issues
When a function is called, the call is matched against definitions to determine what code is to be executed. What happens if the call has a different number of parameters to the definition?
For example, suppose that { open door } is defined (it causes the door to open) and { open } is also defined (it displays an error message saying "You didnt tell me what it was you wanted to open"). Someone wants to OPEN DOOR WITH KEY. What happens?
The general rule is that function calls which match partial definitions will continue until the parameters run out. This means that although [ open door key ]() has a spurious third parameter, it will match { open door } before { open }. A call such as [ open box key ]() would match { open }, because BOX is not a kind of DOOR (I trust!).
The opposite does not apply. If only { open door } were defined and the call made was [ open ](), it would not execute the code for { open door }.
Parameter Naming
Lets say that someone wants to open a particular door, perhaps DOOR12. The call [ open door12 ]() would match { open door }. When the associated code gets to run, however, how does it know you meant DOOR12 and not the general concept of DOOR? If it opens DOOR then all doors inheriting their default state from DOOR will be opened!
What you do is name the parameters. The long way is like C does it, by giving parameters identifiers: { open door d }. The short way is to give all parameters implicit identifiers, as if they were defined as e.g. { open zeroth door first }. To refer to the door that instantiates the first parameter, youd use first. In MUD2, I implemented both explicit and implicit naming, but found that I only ever used the implicit form.
Its important that you can access what a parameter is bound to, rather than what it is named.
Data Types
Where do things like integers and strings fit into this?
You pre-build their classes into the atom hierarchy. All integers are instances of the class INTEGER, all strings are a STRING, all lists are a LIST and so on. When pattern-matching, you do class membership on the fly (you dont have to load all integers into the hierarchy, thank goodness just make sure that if you have an integer then it matches the INTEGER class).
The MUD2 atom hierarchy is pre-loaded with basic data types as follows:
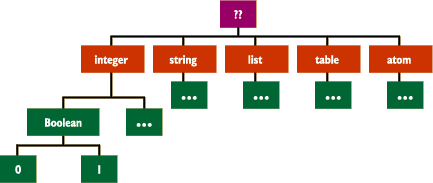
All integers go under INTEGER, all new atoms go under ATOM. BOOLEAN is complete in itself, LIST gives the capacity to produce basic data structures and TABLE is a kind of portable vector/switch thats only slightly less trouble than its worth...
Objects as Functors
Although in general youll use action atoms for the zeroth parameter of a definition and object atoms for the rest, you can mix and match. An example is where you might want to use a player name as a verb.
Lets have a diadic operator, !!, which takes an atom on the left and a string on the right. It transmits the string to the terminal attached to the atom (if there is one). In other words, it sends messages to players. We can implement a simple TELL function quite easily:
{ tell player string }: first !! second
However, we can also let people omit the TELL:
{ player string }: zeroth !! first
In this example, someone is typing FRED "HELLO" rather than TELL FRED "HELLO", but both work. The player name, FRED, is an object in the first case and a functor in the second. Kinda neat, huh? Hey, Just a Moment..!I just said that if someone types FRED "HELLO" then that invokes [ fred "hello" ](). If they type TELL FRED "HELLO" then that invokes [ tell fred "hello" ](). Isnt that taking rather a lot for granted? What if theyd typed TELL EVERYONE EXCEPT FRED "HELLO"?
In my next article Ill be starting a new topic: parsing.
Recent Discussions on Notes from the Dawn of Time:
|
|
 |